Modern web apps frequently use the interactive feature drag and drop. It enables users to drag objects to different locations on the screen by clicking and holding them with the mouse. When automating online apps, it’s crucial to verify the drag and drop functionality.
In this blog, we’ll look at how to use Java and Selenium to automate the Drag and Drop feature.
Understanding The Drag and Drop Process in Selenium
We must understand the operation of drag and drop before we can automate those actions in our web application. Events are triggered in the browser when you drag and drop element:
- Mousedown Event:
A mousedown event is triggered when you click on an element while you keep holding down the mouse button. The drag operation has started in this event state. - Mousemove Event:
A mousemove event is triggered whenever the mouse is moved while the mouse button is depressed. While the mouse is moved, this event is continuously fired and provides the mouse pointer’s coordinates. - Mouseup Event:
A mouseup event is triggered when you release the mouse button. This event indicates that the drag operation is finished.
The browser may also trigger additional events, like dragstart, dragenter, dragover, and drop, during a drag-and-drop operation. Both the source and destination elements as well as the full web page get these events.
It is challenging to automate Drag and Drop using Selenium due to the asynchronous nature of the events fired during a Drag and Drop operation. The Actions class of Selenium WebDriver can be used to handle this.
Implementing Drag and Drop with Selenium Action Class
In the following section, you will see how you can use the Actions class to automate Drag and Drop actions in Selenium WebDriver.
- Locate the source and target elements:
While automating Drag and Drop functionality the first step you have to perform is, locating the Source element(the element that will be dragged) and the Target element(the element where the source element will be dropped).
Syntax:
WebElement source = driver.findElement(By.id("source"));
WebElement target = driver.findElement(By.id("target"));
- Create an Actions object:
After locating the source and target elements you need to create an object of the Actions class. Actions class provides a way to chain together multiple actions, such as moving the mouse and clicking on an element.
Syntax:
Actions actions = new Actions(driver);
- Move the source element:
After creating an object of the Action class, you need to move the mouse pointer to the Source element using the moveByOffset() or moveToElement() methods.
Syntax:
actions.moveToElement(source)
- Click and hold the source element:
Once you move the mouse pointer to the source element, you need to click and hold the element using the clickAndHold() method.
Syntax:
actions.moveToElement(source).clickAndHold()
- Move the source element to the target element:
While holding the mouse button, you need to use moveToElement() method to move Source element to the Target element.
Syntax:
actions.moveToElement(source).clickAndHold().moveToElement(target)
- Release the source element:
Once the source element has been moved to the target element, you need to release Source element by calling the release() method.
Syntax:
actions.moveToElement(source).clickAndHold().moveToElement(target).release()
Practical Demonstration of Drag and Drop In Selenium
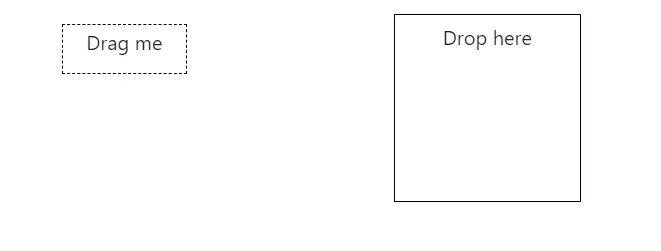
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class DragAndDropExample {
public static void main(String[] args) {
// Create a new instance of the Chrome driver
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
// Navigate to the website
driver.get("https://demoqa.com/droppable/");
// Find the source element and target element for the drag and drop operation
WebElement source = driver.findElement(By.id("draggable"));
WebElement target = driver.findElement(By.id("droppable"));
// Create an instance of the Actions class
Actions actions = new Actions(driver);
// Perform the drag and drop operation
actions.clickAndHold(source).moveToElement(target).release(target).build().perform();
// Wait for the target element to change
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(ExpectedConditions.textToBePresentInElement(target, "Dropped!"));
String Droptext = target.getText();
if(Droptext.equals("Dropped!"))
{
System.out.println("Successfully Dropped");
}
else
{
System.out.println("Dropp Failed");
}
// Close the browser
driver.quit();
}
}
Output:

With the above implementation, you can easily automate Drag and Drop actions in your web application. However, some Drag and Drop scenarios can be more complex, such as target element is not visible on the screen or when the source element needs to be dropped at a specific location.
Advanced Drag and Drop Techniques with Selenium WebDriver
In above section, we have covered the basics technique of automating Drag and Drop functionality. In this section, we will explore some of the advanced Drag and Drop techniques you can use with Selenium.
- Dragging to an offset:
For Dragging and Dropping the Source element onto the center of the Target element you need to use dragAndDrop() method, which is the default method of the Actions class. If you want to drop the source element at a specific location, you can use dragAndDropBy() method instead of default method.
This method basically takes two arguments:
i.e: The source element and x, y offset.
Syntax:
actions.dragAndDropBy(source, x, y).perform();
Practical Demonstration of Dragging to an offset:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
public class AdvancedDragAndDropExample {
public static void main(String[] args) {
// Set up the driver and open the web page
WebDriver driver = new ChromeDriver();
// Navigate to the website
driver.get("https://demoqa.com/droppable/");
// Locate the source and target elements
WebElement source = driver.findElement(By.id("draggable"));
WebElement target = driver.findElement(By.id("droppable"));
// Create an object of the Actions class
Actions actions = new Actions(driver);
// Dragging to an offset
actions.dragAndDropBy(source, 100, 50).perform();
// Close the browser
driver.quit();
}
}
- Dragging to an element on the page:
In some cases, the target element may not be visible on the screen, or you may need to drop the source element onto another element. To do this, you can use the moveToElement() method to move the mouse pointer to another element on the page.
Syntax:
actions.moveToElement(target).perform();
actions.dragAndDrop(source, target).perform();
Practical Demonstration of Dragging to an element on the page:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
public class AdvancedDragAndDropExample {
public static void main(String[] args) {
// Set up the driver and open the web page
WebDriver driver = new ChromeDriver();
// Navigate to the website
driver.get("https://demoqa.com/droppable/");
// Locate the source and target elements
WebElement source = driver.findElement(By.id("draggable"));
WebElement target = driver.findElement(By.id("droppable"));
// Create an object of the Actions class
Actions actions = new Actions(driver);
// Dragging to an element on the page
actions.moveToElement(target).perform();
actions.dragAndDrop(source, target).perform();
// Close the browser
//driver.quit();
}
}
- Dragging between two windows or iframes:
When you want to drag and drop between two windows or iframes, before performing the Drag and Drop action you may need to switch to the window or iframe where you want to drop the element. You can do this using the switchTo() method of WebDriver.
Syntax:
//switch to iframe
driver.switchTo().frame(0);
//switch to window
driver.switchTo().window(handle);
Practical Demonstration of Dragging between two windows or iframes:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
public class AdvancedDragAndDropExample {
public static void main(String[] args) {
// Set up the driver and open the web page
WebDriver driver = new ChromeDriver();
driver.get("https://demoqa.com/droppable/");
// Locate the source and target elements
WebElement source = driver.findElement(By.id("draggable"));
WebElement target = driver.findElement(By.id("droppable"));
// Create an object of the Actions class
Actions actions = new Actions(driver);
// Dragging between two windows or iframes
driver.findElement(By.id("new-tab-button")).click();
for (String handle : driver.getWindowHandles()) {
driver.switchTo().window(handle);
}
driver.get("https://demoqa.com/droppable/");
WebElement newTarget = driver.findElement(By.id("droppable"));
actions.dragAndDrop(source, newTarget).perform();
driver.close();
driver.switchTo().window(driver.getWindowHandles().iterator().next());
// Close the browser
driver.quit();
}
}
Troubleshooting Common Issues with Drag and Drop
Drag and Drop can sometimes become difficult and may not work as expected. In this section, we will discuss some common issues that can occur and how to troubleshoot them.
- Element not interactable:
If you receive an exception of “element not interactable” while performing a Drag and Drop operation. This may happen because the element is not visible, enabled, or not in the correct state. You can try adding a wait condition for the element to become visible or enabled before attempting the Drag and Drop operation.
Syntax:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id("element-id")));
- Drag and drop not working:
If your Drag and Drop operation is not working at all. It may happen because the element is not draggable. You can check this by inspecting the element and looking for the draggable attribute. If the attribute is not present on the web page, you will need to use JavaScript executor to make the element draggable.
Syntax:
WebElement element = driver.findElement(By.id("element-id"));
Boolean isDraggable = (Boolean) ((JavascriptExecutor) driver).executeScript("return arguments[0].hasAttribute('draggable');", element);
if (isDraggable) {
Actions actions = new Actions(driver);
actions.dragAndDrop(source, target).perform();
}
- Drag and drop offset issues:
If the element is not being dropped at the correct location, it may happen because of an offset issue. You need to use the dragAndDropBy() method to add an offset to the element before dropping it. You can also try using the moveByOffset() method to move the cursor to the correct location before dropping the element.
Syntax:
Actions actions = new Actions(driver);
//dragAndDropBy()
actions.dragAndDropBy(source, xOffset, yOffset).perform();
//moveByOffset()
actions.moveToElement(element, xOffset, yOffset).clickAndHold().moveByOffset(50, 50).release().perform();
- Drag and drop not working in iframes:
If your Drag and Drop operation is not working inside an iframe, you need to switch to the iframe before performing the operation. You can do this using the switchTo().frame() method of the WebDriver class.
Syntax:
driver.switchTo().frame("iframe-name");
WebElement element = driver.findElement(By.id("element-id"));
Actions actions = new Actions(driver);
actions.dragAndDrop(source, target).perform();
driver.switchTo().defaultContent();
- Drag and drop not working in different browsers:
If your Drag and Drop operation is not working in different browsers, it may be because of Browser Specific issues. Try updating your browser or using a different browser to see if the issue keeps on showing.
Syntax:
WebDriver driver = new FirefoxDriver();
// or
WebDriver driver = new ChromeDriver();
// or
WebDriver driver = new EdgeDriver();
// etc.
These are just some examples of how you can troubleshoot common issues with Drag and Drop using Java and Selenium. The specific syntax/code will depend on the specific issue you’re experiencing.
Conclusion
In conclusion, Drag and Drop functionality is a common feature that is often required for testing modern web applications. With the techniques and tips outlined in this article, you should be well prepared to implement and troubleshoot Drag and Drop functionality with Selenium WebDriver in your own testing projects.
Remember to always keep your testing environment up-to-date and take advantage of the latest features and tools available. By following best practices and staying on top of new developments in automation testing, you can ensure that your testing efforts are efficient, effective, and accurate.
What is drag and drop functionality in web applications?
Drag and drop allows users to click and hold on an object, move it to a new location, and release the mouse button to drop the object in the new location. It’s a common feature in modern web applications that enhances the user experience.
How do I implement drag and drop with Selenium WebDriver?
You can use the Actions class in Selenium WebDriver to simulate drag and drop actions. You can also use the clickAndHold(), dragAndDrop(), and moveByOffset() methods to move an element in small increments, and the release() method to release the mouse button.
What are some common issues with drag and drop in Selenium WebDriver?
Some common issues with drag and drop in Selenium WebDriver include incorrect offsets, issues with drag and drop across iframes, and compatibility issues with specific browsers or web applications.