In web automation, dropdowns play a vital role in testing and simulating user interactions. Automating the selection of option(s) from a list, saves time and reduces manual effort. Handling dropdowns in Selenium is essential for testing web applications as it enables you to verify that the dropdown options are working as expected.
- What is Dropdown?
- Types of Dropdown in Selenium
- How to Identify Dropdown in Selenium
- How to Handle Dropdown in Selenium Using Select Class
- How to Handle Dropdown in Selenium Without using Select Class
- How to Handle Dynamic Dropdown in Selenium
- How to Handle Auto-Suggestion Dropdown in Selenium
- Best Practices for Handling Dropdown in Selenium
- Conclusion
- FAQ
What is Dropdown?
A dropdown is an element on the web pages that allows us to choose an option from a list of options. It consists of a clickable element, that is when the button is clicked, it displays a list of options that we can choose from. Dropdown is commonly used element on web pages for various reasons such as selecting a language, choosing a country, or filtering search results.
Below are some examples of dropdown that we might see on web pages:
- Language Selector Dropdown:
This type of dropdown allows us to choose the language in which we want the website content to be displayed. It provides options for the selection of languages, such as English, Hindi, Marathi, and so on. - Country Selector Dropdown:
This dropdown allows us to select either the country we currently reside in or the country we want to search for. It normally includes a list of country names, flags, and sometimes a search box to help us in finding our preferred country. - Filter Dropdown:
This dropdown is commonly used on E-Commerce websites. It allows us to filter search results based on required criteria such as price range, brand, color, size, etc… - Date Selector Dropdown:
This dropdown allows us to choose a date from a calendar box. - Time Selector Dropdown:
This dropdown allows us to select a specific time from a list of times or a clock interface.
Types of Dropdown in Selenium
In Selenium, we commonly encounter three types of dropdowns, which are described as follows:
- Static Dropdown:
Static Dropdown is a dropdown that contains a fixed set of options defined in the HTML code and does not change during runtime. As the options are fixed, selecting them is relatively easy in automation testing. we can you following methods to automate the selection of static dropdowns. - Dynamic Dropdown:
Dynamic Dropdown is a dropdown that contains options that may change based on certain conditions, such as user input or server side data changes. Additional steps may be required to populate the dropdown, and the options may not be available until the page finishes loading. To handle dynamic dropdowns, we need to use explicit wait in our automaton script. - Auto suggestion Dropdown:
Auto suggestion Dropdown is a dropdown that provides suggestions to us based on our input. As we type something, the dropdown dynamically displays a list of suggestions related to our input.
Populating the dropdown may require additional steps, and the options may not be available until the page finishes loading. To handle auto suggestion dropdowns, we can use sendKeys() method to enter the input, and for selecting any of the suggestion from the suggestions list we can use the Actions class in selenium.
How to Identify Dropdown in Selenium
Identifying dropdown elements in Selenium is a critical step in handling them effectively during automation testing. There are several Locators we can use to identify dropdown in Selenium, including:
- By ID
- By Name
- By Class Name
- By Tag Name
- By cssSelector
- By Xpath
Read Our Blog on Web Locators In Selenium for a step-by-step guide on how to use different types of WebLocators to find any elements on the page.
How to Handle Dropdown in Selenium Using Select Class
The Select class in Selenium is a powerful tool for handling dropdowns in automation testing. It provides a simple and effective way to interact with dropdown elements and select options based on visible text, value, or index.
In this section, we will discuss how to handle dropdowns in Selenium using the Select class.
I. Creating a Select Object:
For using the select class in selenium, we must first need to create a Select object for the dropdown element. We can create that by locating the dropdown element using any of the locators mentioned in the above section(How to Identify Dropdown in Selenium) and passing it as a parameter to the Select constructor.
Syntax:
WebElement dropdownElement = driver.findElement(By.id("dropdown"));
Select dropdown = new Select(dropdownElement);
II. Selecting Options:
Once we have created a Select object for the dropdown element, we can then select an option from the list of options using one of the following methods:
- By Visible Text:
To select an option based on its visible text, we can use the selectByVisibleText() method.
Syntax:
dropdown.selectByVisibleText("Option 1");
- By Value:
To select an option based on its value attribute, we can use the selectByValue() method.
Syntax:
dropdown.selectByVisibleText("Option 1");
- By Index:
To select an option based on its index, we can use the selectByIndex() method.
Syntax:
dropdown.selectByValue("value1");
III. Getting Selected Options:
For getting the selected option(s) from the dropdown, we can use the getAllSelectedOptions() or getFirstSelectedOption() method.
Syntax:
List<WebElement> selectedOptions = dropdown.getAllSelectedOptions();
WebElement firstSelectedOption = dropdown.getFirstSelectedOption();
IV. Getting All Options:
For getting all the options from the dropdown, we can use the getOptions() method.
Syntax:
List<WebElement> options = dropdown.getOptions();
V. Deselecting Options:
If our web page is using a Multi Selection dropdown, then we can use one of the following methods to deselect any of the selected option(s) from the dropdown list:
- By Visible Text:
To deselect an option based on its visible text, we can use the deselectByVisibleText() method.
Syntax:
dropdown.deselectByVisibleText("Option 1");
- By Value:
To deselect an option based on its value attribute, we can use the deselectByValue() method.
Syntax:
dropdown.deselectByValue("value1");
- By Index:
To deselect an option based on its index, we can use the deselectByIndex() method.
Syntax:
dropdown.deselectByIndex(0); //deselects the first option
- Deselect All:
To Clear all the selected options, we can use the deselectAll() method.
Syntax:
dropdown.deselectAll();
Practical Demonstration of How to Handle Dropdown in Selenium Using Select Class
We will use http://the-internet.herokuapp.com/dropdown website as an example.

import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.Select;
public class DropdownSelectExample {
public static void main(String[] args) {
// Create a new instance of the ChromeDriver
WebDriver driver = new ChromeDriver();
// Navigate to the website
driver.get("http://the-internet.herokuapp.com/dropdown");
// Locate the dropdown element by ID
WebElement dropdown = driver.findElement(By.id("dropdown"));
// Create a new Select object
Select select = new Select(dropdown);
// Select the second option by visible text
select.selectByVisibleText("Option 2");
// Close the browser
driver.quit();
}
}
Output:

How to Handle Dropdown in Selenium Without using Select Class
In this section, we will discuss how to handle Dropdowns in Selenium without using the Select class.
I. Using click() and sendKeys() methods:
We need to use the click() method, to click the dropdown element that will open the dropdown list.
For selecting any of the option from the options list we need to use sendkeys() method.
We can use this approach for simple dropdowns, but it can be unreliable for more complex dropdowns as there is a chance of getting errors.
Syntax:
WebElement dropdown = driver.findElement(By.id("Dropdown"));
dropdown.click();
dropdown.sendKeys("Option 1");
II. Using Actions class to move to the dropdown and select options:
The Actions class provides a way to perform complex user interactions, including moving the mouse cursor to the dropdown field and clicking on elements. This can be useful for handling dropdowns that require a mouse hover action to open or complex dropdowns with nested elements.
Syntax:
WebElement dropdown = driver.findElement(By.id("Dropdown"));
Actions actions = new Actions(driver);
actions.moveToElement(dropdown).click().sendKeys("Option 1").build().perform();
- moveToElement() method moves the mouse cursor to the dropdown element.
- click() method clicks on the element to open it.
- sendKeys() method selects the desired option.
Practical Demonstration of How to Handle Dropdown in Selenium without Using Select Class
We will use https://demo.seleniumeasy.com/basic-select-dropdown-demo.html website as an example.

import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
public class DropdownwithoutSelectExample {
public static void main(String[] args) {
// Create a new instance of the ChromeDriver
WebDriver driver = new ChromeDriver();
// Navigate to the website
driver.get("https://demo.seleniumeasy.com/basic-select-dropdown-demo.html");
// Find the dropdown element by its ID
WebElement dropdown = driver.findElement(By.id("select-demo"));
// Click on the dropdown to expand its options
dropdown.click();
// Find the option element with the desired value and click on it
WebElement option = driver.findElement(By.xpath("//option[@value='Thursday']"));
option.click();
//Printing the selected value in console
String[] SelectedValue = driver.findElement(By.cssSelector(".selected-value")).getText().split("-");
System.out.println("Day Selected:" +SelectedValue[1]);
// Close the browser
driver.quit();
}
}
Output:

How to Handle Dynamic Dropdown in Selenium
In this section, we will discuss how to handle Dynamic Dropdowns in Selenium.
I. Identifying dynamic dropdown elements:
To identify a dynamic dropdown element,we need to inspect the HTML code of the web page and look for elements that change when we interact with them. We can use any of the locators mentioned in the section (How to identify dropdowns in Selenium) to locate the element.
II. Using explicit waits to wait for dropdown options to load:
As dynamic dropdowns may take some time to load their options, we need to use explicit waits to wait for them to become available. We can use the WebDriverWait class to wait for the element to be clickable, visible, or to have a certain attribute or property.
Syntax:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement dynamicDropdown = wait.until(ExpectedConditions.elementToBeClickable(By.id("DynamicDropdown")));
III. Selecting options from dynamic dropdowns:
Once the dynamic dropdown options are loaded, we can use the same methods to select options as we used for static dropdowns.
(How to Handle Dropdowns in Selenium Using Select Class)
Syntax:
Select dropdown = new Select(driver.findElement(By.id("DynamicDropdown")));
dropdown.selectByVisibleText("Option 1");
Practical Demonstration of How to Handle Dynamic Dropdown in Selenium
We will use https://www.spicejet.com/ website as an example.
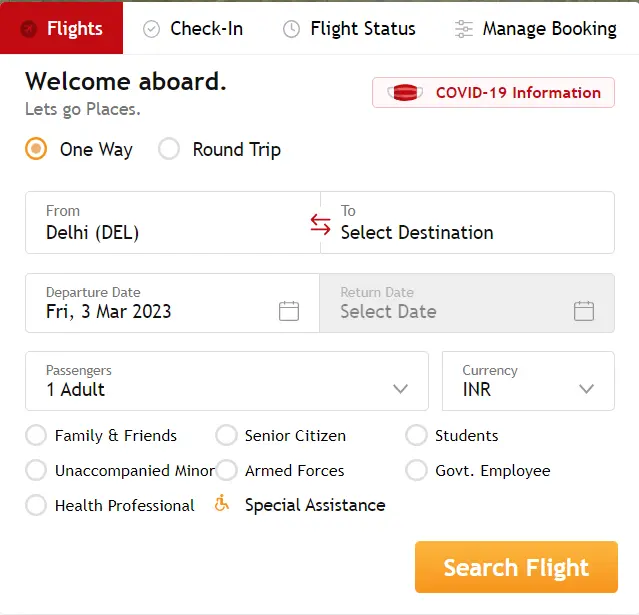
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class DynamicDropdownExample {
public static void main(String[] args) {
// Create a new instance of the ChromeDriver
WebDriver driver = new ChromeDriver();
// Navigating to the website
driver.get("https://www.spicejet.com/");
// Selecting "From" city
driver.findElement(By.xpath("//div[@data-testid='to-testID-origin']")).click();
driver.findElement(By.xpath("//div[text()='BOM']")).click();
// Using explicit wait to wait for "To" city dropdown to be visible
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement toCityDropdown = wait.until(ExpectedConditions
.visibilityOfElementLocated(By.xpath("//div[contains(text(),'Select a region and city below')]")));
// Selecting "To" city
driver.findElement(By.xpath("//div[@data-testid='to-testID-destination'] //div[text()='DEL']")).click();
// Selecting "Departure Date"
driver.findElement(By.xpath("//div[contains(@class,'r-y47klf ')]")).click();
// Selecting "Passengers" dropdown
driver.findElement(By.xpath("//div[@data-testid='home-page-travellers']")).click();
// Selecting "Adults" option
for (int i = 1; i < 3; i++) {
driver.findElement(By.xpath("//div[@data-testid='Adult-testID-plus-one-cta']")).click();
}
driver.findElement(By.xpath("//div[@data-testid='home-page-travellers-done-cta']")).click();
// Clicking "Search" button
driver.findElement(By.xpath("//div[@data-testid='home-page-flight-cta']")).click();
// Closing the browser
driver.quit();
}
}
Output:
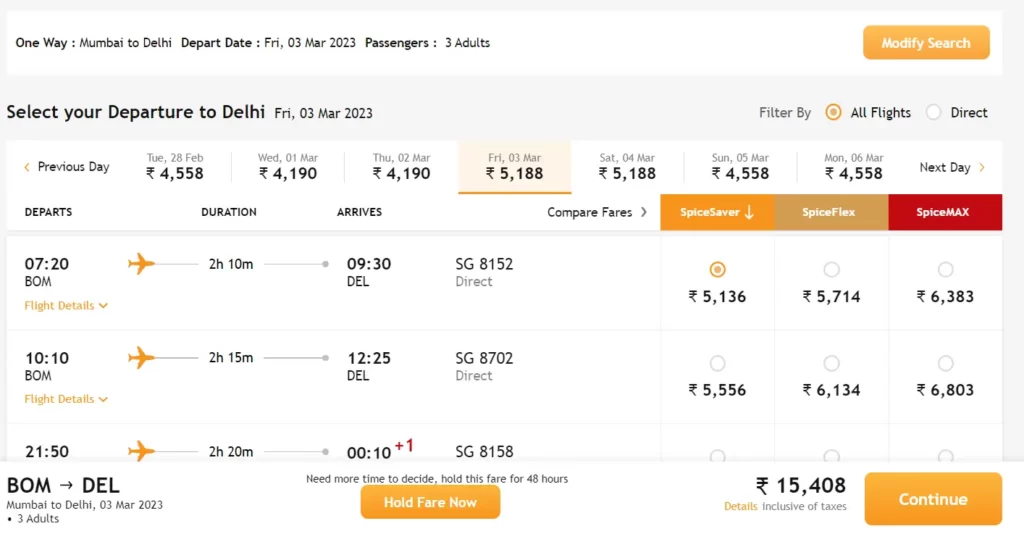
How to Handle Auto-Suggestion Dropdown in Selenium
In this section, we will discuss how to handle Auto-Suggestion Dropdowns in Selenium.
I. Identifying auto suggestion dropdown elements:
Firstly, we need to identify the input field element that triggers the auto suggestion dropdown. We can use the same methods that we used for identifying static dropdown elements(How to identify dropdowns in Selenium).
Syntax:
WebElement inputField = driver.findElement(By.id("inputFieldId"));
II. Sending keys to the input field to trigger suggestions:
Once we have identified the input field element, we can use the sendKeys() method to enter some text in the input field. This will trigger the auto suggestion dropdown with a list of suggestions.
Syntax:
inputField.sendKeys("selenium");
III. Using explicit waits to wait for dropdown options to load:
As auto suggestion dropdowns may take some time to load their options, we need to use explicit waits to wait for them to become available. We can use the WebDriverWait class to wait for the element to be visible.
Syntax:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement suggestionList = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("suggestionListId")));
IV. Clicking on a suggestion to select it:
After the auto suggestion dropdown is displayed, we can use findElements() method to locate the suggestion elements list, and then we need use the click() method for selecting the option from the suggestions list.
Syntax:
// Find all the suggestion elements
List<WebElement> suggestions = suggestionList.findElements(By.tagName("li"));
// Click on the second suggestion
suggestions.get(1).click();
Practical Demonstration of How to Handle Auto-Suggestion Dropdown in Selenium
We will use https://www.google.com/ website as an example.
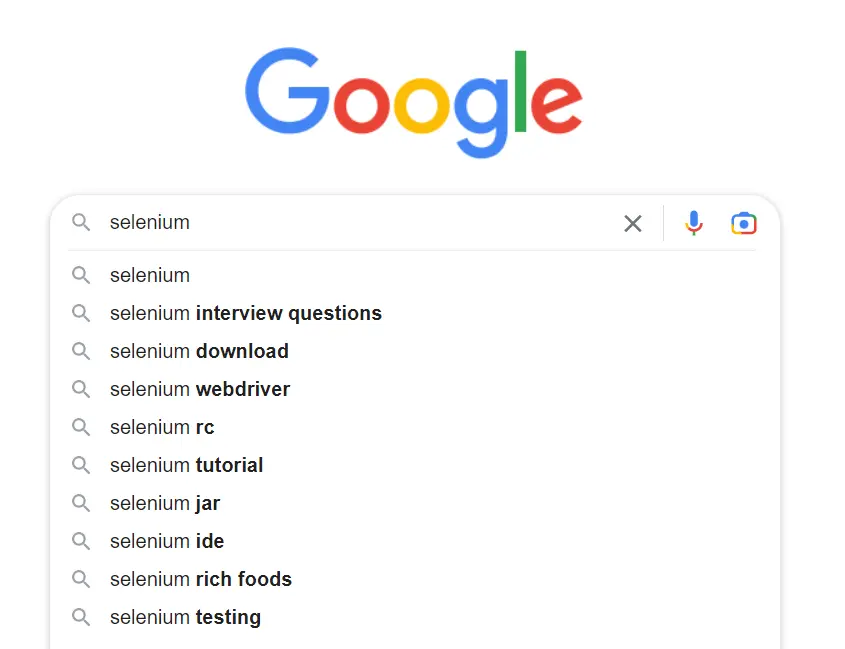
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import java.time.Duration;
import java.util.List;
public class AutoSuggestionDropdownExample {
public static void main(String[] args) {
// Launch the Chrome browser
WebDriver driver = new ChromeDriver();
// Navigate to the Google home page
driver.get("https://www.google.com/");
// Find the search input element and send keys to it
WebElement searchInput = driver.findElement(By.name("q"));
searchInput.sendKeys("Selenium");
// Wait for the auto-suggestion dropdown to appear and get its elements
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
By suggestionsLocator = By.xpath("//div[@class='mkHrUc']//li");
wait.until(ExpectedConditions.visibilityOfElementLocated(suggestionsLocator));
List<WebElement> suggestions = driver.findElements(suggestionsLocator);
// Click on the first suggestion
suggestions.get(0).click();
// Close the browser
driver.quit();
}
}
Output:
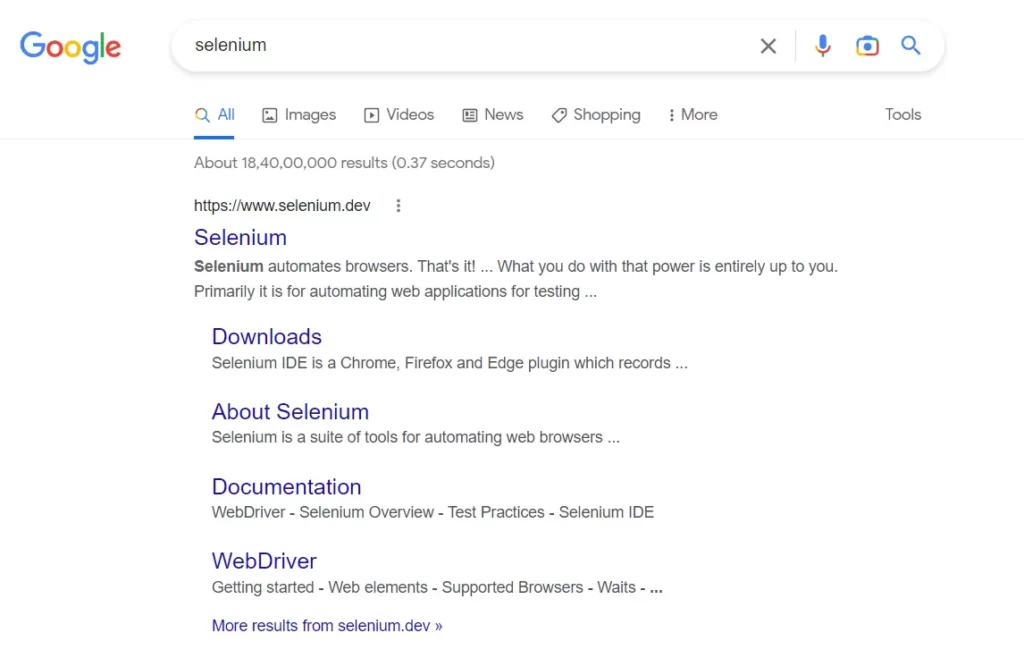
Best Practices for Handling Dropdown in Selenium
When it comes to handling dropdowns in Selenium, there are a few best practices that we should keep in mind to ensure that our tests are efficient, reliable, and maintainable.
- Use explicit waits to wait for dropdowns to load:
Dropdowns may take some time to load on a web page, particularly if they are dynamic or auto suggestion dropdowns. Explicit waits can help us to make sure that the dropdown is fully loaded before we try to interact with them. - Verify that the expected dropdown options are present:
Before interacting with the dropdown, make sure that the expected options are present. This will help us to find any unexpected changes to the dropdown options that may cause our tests to get fail. - Test different scenarios (selecting/deselecting options, selecting invalid options, etc.):
It is important to test different scenarios to make sure that the dropdown is working as expected. Test selecting/deselecting options, selecting invalid options, and other scenarios that may be related to our application.
Conclusion
In conclusion, dropdowns are an important element on web pages, and handling them effectively is crucial for web automation testing. In this blog post, we have covered different types of dropdowns and how to identify and handle them using Selenium and java. We discussed the use of the Select class and alternative methods for handling dropdowns, such as using click() and sendKeys() methods, and the Actions class. By mastering dropdown handling in Selenium, you can improve the accuracy and effectiveness of your web automation testing.
FAQ
What are dropdowns in web automation?
Dropdowns are interactive UI elements that display a list of options that can be selected by the user. They are commonly used in web applications and can be automated using Selenium.
What is the difference between static and dynamic dropdowns in Selenium?
Static dropdowns have a fixed set of options, while dynamic dropdowns have options that can change based on other user actions or data inputs.
Why is handling dropdowns important in Selenium automation?
Dropdowns are a common element on web pages and can impact the user experience, so properly testing and handling them in Selenium ensures that the website/application is functioning as expected.