Selenium is a popular open-source tool for website automation, it can significantly reduce the time and effort required for manual testing. One of the most important aspects of Selenium testing is mastering the use of waits, which allows you to wait for specific conditions to be met before continuing with your test automation script.
In this blog post, we’ll explore the different types of waits available in Selenium using Java, and we will provide you with live examples that demonstrate how to use waits effectively.
What are Waits in Selenium?
Waits are a mechanism in Selenium that allows you to wait for a specific condition to be met, before moving forward with the execution of your automation script. It is important because web applications can often have dynamic content that takes time to load, such as Ajax calls or javascript animations, which can create issues while you are trying to automate any module.
Types of Waits in Selenium?
There are mainly three types of waits available in Selenium(Implicit Wait, Explicit Wait, and Fluent Wait). Each type of wait has its own benefits and functionality, which we will discuss in the following sections:
I. Implicit Wait
Implicit Wait is a type of wait in Selenium that allows you to set a default wait time for the entire script. When you use Implicit Wait, Selenium will wait for a time that you have specified in your automation script before trying to locate an element on the web page.
The main benefit of Implicit Wait is, it is easy to use and can be set once for the entire automation script, which can save your lots of time and effort.
The disadvantage of Implicit Wait is that if you set too high wait time, it can slow down your automation script.
For Implicit Wait, you can use the implicitlyWait() method, which is part of the WebDriver class in selenium.
Here is a syntax of Implicit Wait that you can use in your automation script:
WebDriver driver = new ChromeDriver();
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
Practical Demonstration of Implicit Wait
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.time.Duration;
public class ImplicitWaitExample {
public static void main(String[] args) {
// Create a new instance of the ChromeDriver
WebDriver driver = new ChromeDriver();
// Set the implicit wait time to 5 seconds
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(5));
// Navigate to the website
driver.get("https://testingmint.com/");
// Find the search box and enter the keyword "selenium"
driver.findElement(By.xpath("(//*[name()='svg'])[15]")).click();
WebElement searchBox = driver.findElement(By.xpath("//input[@title='Search']"));
searchBox.sendKeys("selenium");
searchBox.submit();
// Wait for the search results to be displayed
WebElement searchResult = driver.findElement(By.cssSelector(".search-results"));
System.out.println(searchResult.getText());
// Close the browser
driver.quit();
}
}
Output:
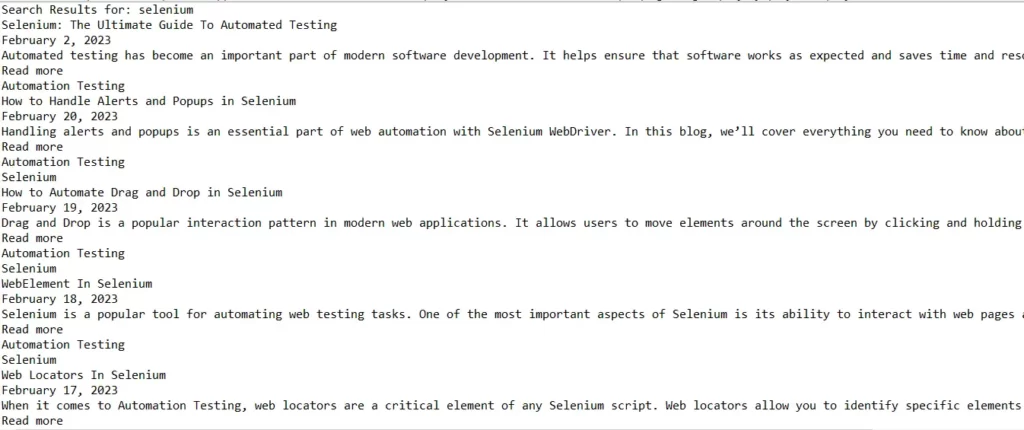
II. Explicit Wait
Explicit Wait is a more detailed type of wait in Selenium. It allows you to wait for a specific condition to be met, before moving forward with the execution of your test automation script. With Explicit Wait, you can set a wait time for a specific element or condition which can improve the efficiency of your script.
For Explicit Wait, you can use WebDriverWait class that allows you to specify a wait time and the condition that you want to wait for.
Here is a syntax of Explicit Wait that you can use in your automation script:
WebDriver driver = new ChromeDriver();
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement button = wait.until(ExpectedConditions.elementToBeClickable(By.id("myButton")));
button.click();
List of Expected Conditions in Explicit Wait
Following is the list of all the expected conditions available in the ExpectedConditions class, which can be used with Explicit Wait in Selenium:
- alertIsPresent():
Waits till the alert gets present. - elementToBeClickable(locator):
Waits for an element to be clickable. - elementToBeSelected(element):
Waits till the element gets selected. - frameToBeAvailableAndSwitchToIt(locator):
Waits for a frame to be available and switches to it. - invisibilityOf(element):
Waits till the element become invisible. - presenceOfAllElementsLocatedBy(locator):
Waits for all elements located by a given locator to be present. - presenceOfElementLocated(locator):
Waits for an element located by a given locator to be present. - textToBePresentInElement(element, text):
Waits till the given text gets present in an element. - textToBePresentInElementLocated(locator, text):
Waits for a given text to be present in an element located by a given locator. - titleIs(title):
Waits for a page title to be a specific value. - titleContains(title):
Waits for a page title to contain a specific value. - visibilityOf(element):
Waits for an element to become visible.
Practical Demonstration of Explicit Wait
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import java.time.Duration;
public class ExplicitWaitExample {
public static void main(String[] args) {
// Create a new instance of the ChromeDriver
WebDriver driver = new ChromeDriver();
// Navigate to the website
driver.get("https://testingmint.com/");
// Find the search box and enter the keyword "selenium"
driver.findElement(By.xpath("(//*[name()='svg'])[15]")).click();
WebElement searchBox = driver.findElement(By.xpath("//input[@title='Search']"));
searchBox.sendKeys("selenium");
searchBox.submit();
// Wait for the search results to be displayed
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement searchResult = wait
.until(ExpectedConditions.visibilityOfElementLocated(By.cssSelector(".search-results")));
System.out.println(searchResult.getText());
// Close the browser
driver.quit();
}
}
Output:
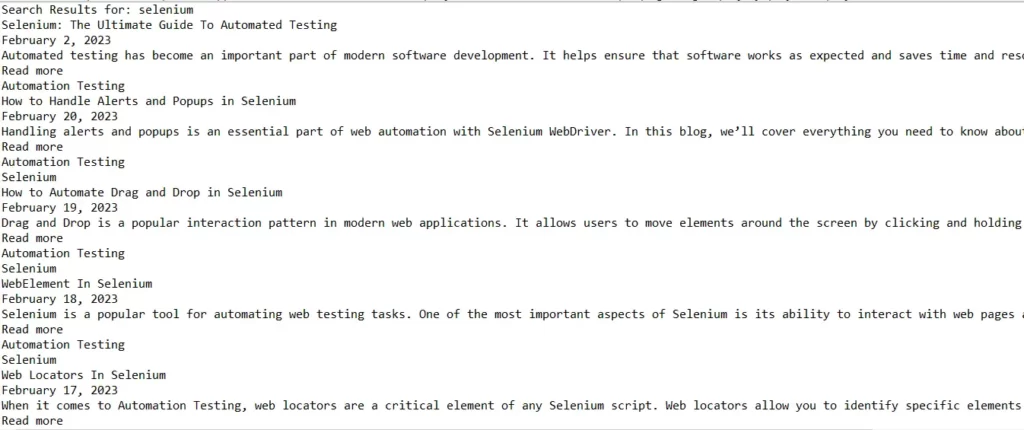
III. Fluent Wait
Fluent Wait is a more flexible type of wait in Selenium and also it can be customizable. It allows you to wait for a specific condition to be met and also how often the request can be made to check the required condition. You can achieve this by setting wait time and polling frequency.
For Fluent Wait, you can use the Wait interface which provides a range of different conditions that you can wait for.
Here’s a syntax of Fluent Wait that you can use in your automation script with a wait time of 10 seconds and a polling frequency of 2 seconds:
WebDriver driver = new ChromeDriver();
Wait<WebDriver> wait = new FluentWait<>(driver)
.withTimeout(Duration.ofSeconds(10))
.pollingEvery(Duration.ofSeconds(2))
.ignoring(NoSuchElementException.class);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("myElement")));
List of Wait Interface in Fluent Wait
The Wait interface in Fluent Wait provides different methods to customize the wait conditions, including:
- withTimeout(Duration timeout):
It sets the amount of time to wait for the expected condition to be true before throwing a TimeoutException. - pollingEvery(Duration interval):
It sets the frequency with which the Fluent Wait checks for the expected condition. - ignoring(ExceptionType exceptionType):
It specifies the types of exceptions that should be ignored during the wait period. - ignoringAll(Collection<Class<? extends Throwable>> exceptionTypes):
It specifies a collection of exceptions that should be ignored during the wait period. - withMessage(String message):
It specifies a custom message to be included with the TimeoutException if the expected condition is not met. - until(Function<? super T, V> isTrue):
It specifies the condition to wait for, whereT
is the input type andV
is the output type. The condition can be a lambda expression or a method reference.
Practical Demonstration of Fluent Wait
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.FluentWait;
import org.openqa.selenium.support.ui.Wait;
import java.time.Duration;
public class FluentWaitExample {
public static void main(String[] args) {
// Create a new instance of the ChromeDriver
WebDriver driver = new ChromeDriver();
// Navigate to the Selenium website
driver.get("https://www.selenium.dev/");
// Click on the "Downloads" link, which appears after a delay
Wait<WebDriver> wait = new FluentWait<>(driver).withTimeout(Duration.ofSeconds(30))
.pollingEvery(Duration.ofSeconds(2)).ignoring(Exception.class);
WebElement downloadLink = wait.until(Driver -> driver.findElement(By.linkText("Downloads")));
downloadLink.click();
// Close the browser
driver.quit();
}
}
Output:
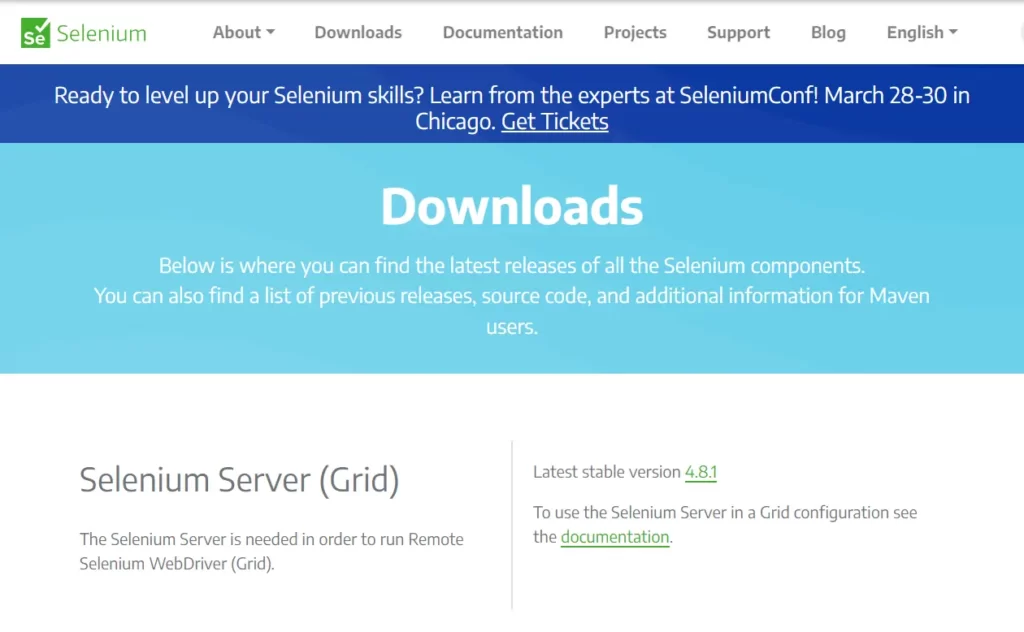
Difference Between Implicit and Explicit Wait
Feature | Implicit Wait | Explicit Wait |
---|---|---|
Scope | Applies to all elements on the page. | Applies only to specific elements. |
Condition | Wait for a fixed amount of time before continuing with the next step. | Wait for a specific condition to be met before continuing with the next step. |
Timeouts | Uses a default timeout value. | Uses a user defined timeout value. |
Flexibility | Less flexible in terms of handling complex conditions. | More flexible in terms of handling complex conditions. |
Control | Less control over wait conditions. | More control over wait conditions. |
Performance | May result in longer wait times and slower test automation. | Can optimize wait times and improve the efficiency of test automation. |
Syntax | driver.manage().timeouts().implicitlyWait (Duration.ofSeconds(seconds)); | WebDriverWait wait = new WebDriverWait(driver, timeOut); |
How to Optimize Waits?
Waits are an essential part of any Selenium test automation script, they can also be a reason behind performance issues if used improperly. Following are some tips for optimizing your waits and improving the efficiency of your test automation
- Use explicit waits instead of implicit waits:
While implicit waits are easier to use, they can result in longer wait times than necessary which can slow down your test automation. Instead of that use explicit waits, which provide more control over when to wait for specific elements. - Use the right conditions for your waits:
The ExpectedConditions class provides a range of different conditions that you can wait for, whether the element is visible or present in web page. Choose the right condition for the element that you are waiting for to minimize the wait time and improve the performance of your script. - Use fluent waits for complex conditions:
If you need to wait for a complex condition such as a file to download or an AJAX request to complete, you can use fluent waits with a polling frequency and a timeout value. This can ensure that your script waits only as long as necessary, while also allowing for some changeability in the time required for the condition to be met. - Use timeouts wisely:
Set the proper value timeout value for your waits, based on the expected time required for the condition to be met. If you set a longer timeout than necessary, it can result in longer wait times and slower automation test. - Use dynamic waits:
In some cases, the time required for a condition to be met may vary, such as when waiting for an element to appear on a web page. In this case, you can use dynamic waits that adjust the timeout value based on the current conditions to optimize the wait times.
Conclusion
In this blog post, we have explored the different types of waits available in Selenium(implicit waits, explicit waits, and fluent waits). We have seen how waits can be used to ensure that your test automation scripts wait for the necessary elements to load, without introducing unnecessary delays.
By mastering the use of waits in Selenium, you can create reliable and accurate test automation scripts that can help you speed up your testing process and catch bugs before they reach production.
We hope that this blog post has provided useful information about waits in Selenium and that it has given you some ideas for how to use waits effectively in your own test automation scripts.
FAQ
What is the purpose of waits in Selenium?
Waits are used to synchronize Selenium commands with the web application under test. By adding waits, you can ensure that the necessary elements and actions are fully loaded and processed before executing the next step of your test script.
How do I optimize my wait times in Selenium?
To optimize your wait times, it is recommended to use the minimum wait time required for the test to pass, avoid using static waits, and use conditional waits to check for specific conditions instead of waiting for a fixed amount of time.
What are the expected conditions available in Selenium?
Selenium provides several expected conditions that can be used with explicit and Fluent waits, including elementToBeClickable
, visibilityOfElementLocated
, titleIs
, presenceOfElementLocated
, and textToBePresentInElement
. These conditions check for specific states or properties of web elements and are useful for waiting for specific events or actions to occur.